Introduction
Manipulating strings efficiently is essential in today’s fast-paced programming world. Python, a versatile and widely used programming language, has various methods for string manipulation, including modifying the case of characters within a string.
This comprehensive guide will delve into the complexities of converting Python strings to lowercase. Whether you are a seasoned Python developer or just beginning your coding journey, this article will provide valuable understandings and applicable examples to master transforming strings to lowercase.
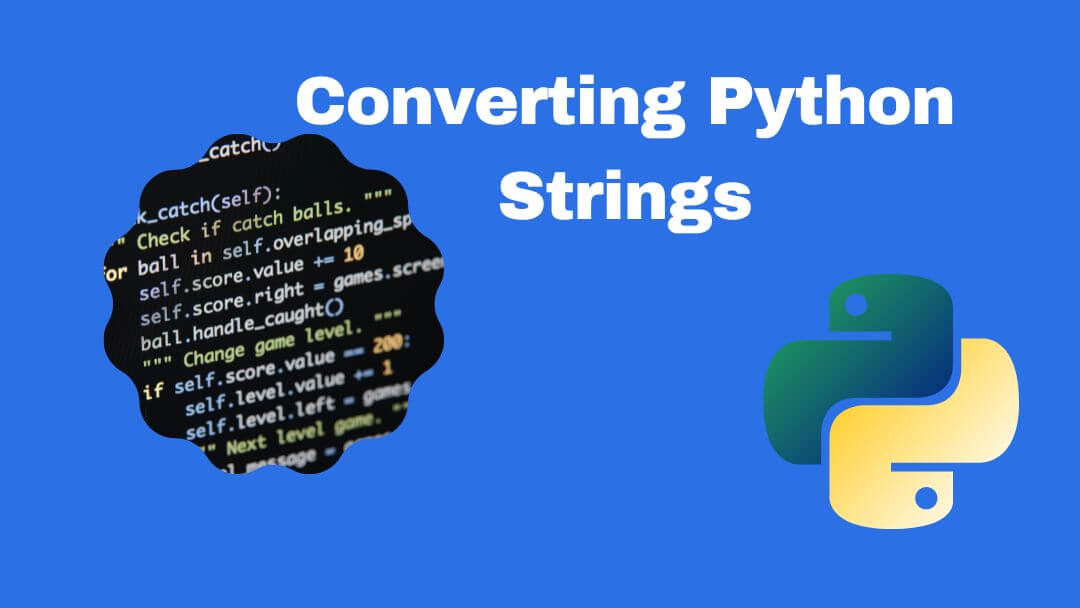
Converting Python Strings
Understanding the Need to Convert to Lowercase
Before we plunge into the methods and techniques of converting Python strings to lowercase, let’s first comprehend why and when you might need to do so.
Data Consistency
In many real-world scenarios, you may encounter data that is not consistently formatted. Imagine a situation where you are processing user input, file contents, or data retrieved from a database.
In these cases, text may be entered in uppercase, lowercase, and combination modes. To ensure data consistency and facilitate further processing or comparison, it is often required to convert all the text to a consistent case, which can be lowercase.
Text Processing and Comparison
Text-based operations, such as searching for a specific word within a larger text or comparing strings for equality, are common tasks in programming.
Converting all text to lowercase simplifies these operations by making them case-insensitive.
This means that “Python,” “python,” and “PYTHON” would all be treated as identical when converted to lowercase, easing the complexity of text manipulation.
User Interface and Display
In applications where text is displayed to users, such as web applications, converting text to lowercase can improve readability and consistency in the user interface. It ensures that headings, labels, and other textual elements appear uniform, enhancing the user experience.
Now that we’ve established the importance of converting text to lowercase, let’s explore the various methods Python offers to achieve this.
Python Methods for Converting to Lowercase
Python provides several built-in methods and functions for converting strings to lowercase. Each method has its advantages and use cases. Let’s examine these methods one by one.
Using the lower() Method
Python’s most straightforward and commonly used method to convert a string to lowercase is the lower() method. This method is available for all string objects and returns a new string with all characters converted to lowercase.
The code
original_string = “Python to lowercase”
lowercase_string = original_string.lower()
print(lowercase_string)
Output:
The code
python to lowercase
The lower() method is simple and efficiently converts the entire string to lowercase. It is suitable for most cases where you must ensure case-insensitivity or consistency in your data.
Using List Comprehension
Another approach to converting a string to lowercase is by using list comprehension. This method allows you to iterate over the characters of the string, convert each character to lowercase, and then join them back into a string.
The code
original_string = “Python to lowercase”
lowercase_string = ”.join([char.lower() for char in original_string])
print(lowercase_string)
Output:
The code
python to lowercase
While this method gives you more control over the conversion process, it is slightly more verbose than the lower() method.
Using the casefold() Method
In addition to the lower() method, Python provides the casefold() method, which performs a more aggressive form of case conversion.
The casefold() method converts characters to lowercase and handles special cases, such as the German ‘ß’ character, making it suitable for case-insensitive comparisons in a broader range of languages.
The code
original_string = “PYTHON tO LoWeRcAsE”
casefolded_string = original_string.casefold()
print(casefolded_string)
Output:
The code
python to lowercase
The casefold() method is particularly useful when working with multilingual text and performing case-insensitive operations.
Handling Unicode Characters
Python’s string manipulation methods, including the ones mentioned above, are Unicode-aware. This means they can handle characters from various scripts and languages, making Python a powerful choice for internationalization and localization tasks.
Conclusion
In this comprehensive guide, we have explored the importance of converting Python strings to lowercase and discussed various methods to accomplish this task. Whether you need to ensure data consistency, simplify text processing, or enhance the user interface, Python provides powerful tools to manipulate strings efficiently.
Remember that choosing the right conversion method depends on your specific requirements. The lower() method is a reliable choice for most cases, while the casefold() method offers additional benefits for handling diverse character sets.
Frequently Asked Questions
1. Why is it important to convert Python strings to lowercase?
Converting Python strings to lowercase is important for several reasons:
- Data Consistency: In real-world scenarios, data may not be consistently formatted. Converting to lowercase ensures uniformity, making data processing and comparison easier.
- Text Processing and Comparison: It simplifies text-based operations by making them case-insensitive, allowing you to search for words or compare strings more effectively.
- User Interface and Display: In applications where text is displayed to users, lowercase text enhances readability and consistency, improving the user experience.
2. When should I use the casefold() method instead of lower() for string conversion?
It would be best if you used the casefold() method when working with multilingual text and when you need to perform case-insensitive operations across a broader range of languages. It handles special cases and characters more comprehensively than the lower() method.
3. How does Python handle Unicode characters when converting strings to lowercase?
Python’s string manipulation methods, including lower(), casefold(), and list comprehension, are Unicode-aware. They can handle characters from various scripts and languages, making Python a powerful choice for internationalization and localization tasks. This ensures that Unicode characters are correctly converted to lowercase while maintaining text integrity.